Okay, I feel like I'm waaay out of my depth here trying to come up with even just the BASIC interpretation of a routine to draw these triangles. Now you're presenting me with
options?!

Right, just so I'm (and the average reader is) clear, these are the three different methods available to draw a filled triangle so far (thanks for chipping in,
@gcewing!):
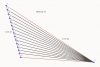
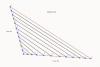
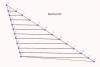
Order of drawing is (mostly) in alphabetical order in those diagrams.
I had an instant reservation pop up as soon as I read about
method #1 - and that's the concern that some pixels might be missed when starting a line from a point on line #1 and a lot of pixels will be overwritten as the 2nd line converges on C. This was a technique used in the 80's and early 90's on 8-bit computers (and even on 16-bit systems in the demo scene) to produce random-ish pretty Moire patterns etc. by taking advantage of the limitations of the graphics systems.
Method #2 looks logical and with less wasted effort (less overwritten pixels - if any). I like this one, but again the concern is how effective would the fill be? For certain angles and triangle sizes, is there a chance pixels could be missed in the fill?
Method #3 looks to have the least pixel overwrites (none) due to rasterising the triangle in a way that is sympathetic to the system it's being drawn on, but as
@BrianHG has pointed out, a diagonal line with an angle less than 45 degrees from horizontal will sometimes have more than one pixel on an edge with the same Y value, creating an overwritten line filling that segment of the triangle. Diagram below shows darker pink lines where multiple horizontal fill lines would overwrite each other:

I'm limited by my understanding of translating all this into BASIC, then HDL, unfortunately. I'm going to have to go away and make a serious effort to understand how the routines are working in the FreeBASIC simulator, but I'm inclined to go with
method #3.